Urban areas all around the world severely suffer due to low air quality, unusual temperature and other factors that puts the health of dwellers at risk. This project was built to monitor and sample atmospheric parameters such as Carbon monoxide concentration, Temperature and Humidity in targeted spots in a city. The collected data was later sampled and analyzed to determine the factors that affects these key parameters. In this project we have documented the data in two spots in an urban population, five kilometers apart from each other. We have analyzed the data and deduced the factors that influenced these results.
Project Brief:
The project is controlled by NodeMCU, an ESP8266 based development board. It collects CO concentration data using MQ07 sensor and monitors temperature and Humidity using DHT11 sensor. Once the data is collected it will be uploaded to cloud ( ThingSpeak platform ) every minute.
We have collected the data in two different places on two different days. The data has been collected in each location for a period of 24 hours. Then we analysed the data to find patterns in CO, temperature and humidity readings. These data patterns helped us to assigned possible causes and derive conclusions.
When NODEMCU uploads the data to cloud it will be time stamped in Thingspeak cloud platform. ThingSpeak will allow us to visualise data in the form of graph so we can monitor the data regularly. Also the uploaded data can be downloaded in any time zone in “CSV” format.
Hardware:
- NodeMCU board
- DHT11( Temperature and Humidity sensor)
- MQ-07(Gas sensor)
- Connecting wires.
Block Diagram:
Circuit Diagram:
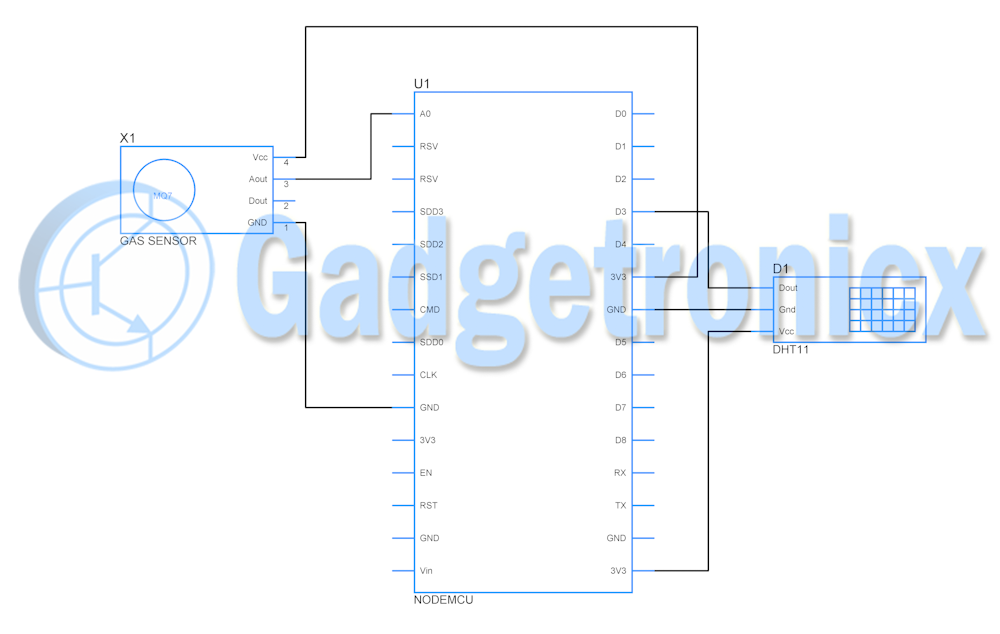
Prototype:
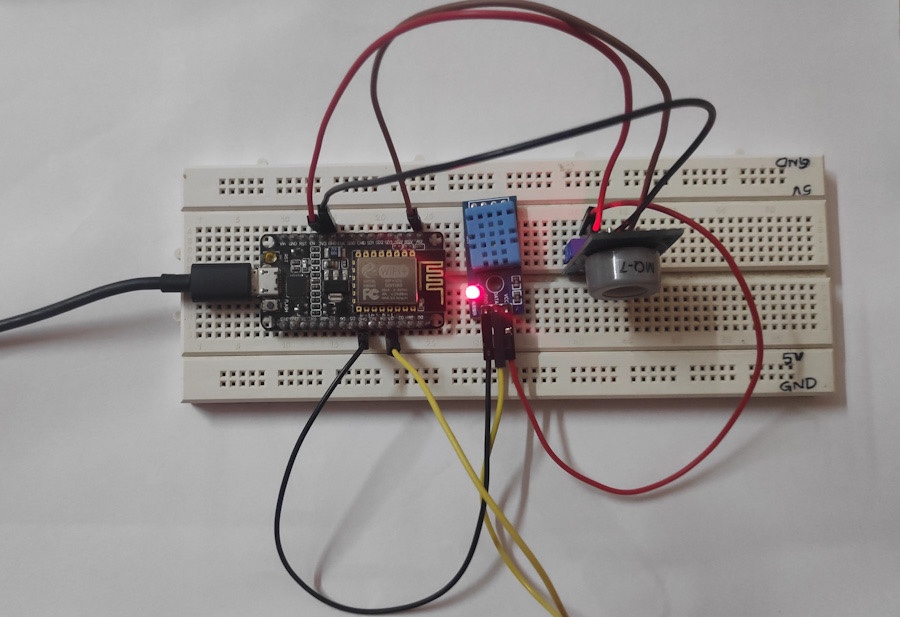
ThingSpeak Dashboard :
Nature of Locations:
We have collected data for 24 hours in two different locations.
- Location 1: It’s an apartment building where it houses about 20 households. Typical urban atmosphere with less trees and moderate pollution from traffic.
- Location 2: Individual house which is compact and tightly packed in a particular locality. Typical urban atmosphere with less trees and moderate pollution from traffic.
Data sets:
The above Excel spreadsheet consists of CO, Temperature and Humidity data. Note that we have experienced problems with DHT11 when collecting data in location 2 henceforth Humidity and temperature has been discarded. Below are some of the interesting observations from these data sets.
- Location 1: Peak in CO levels due to cooking
2. Location 1: Humidity goes high due to rain in night along with drop in temperature
3. Location 2: High CO levels due to cooking
4. Location 2: Outdoor air has less CO levels than indoor air
Conclusions:
From the below data set we can deduce the following observations.
- The CO levels in both the locations went to peak levels during morning to afternoon. This is due to the household cooking where LPG gases are used. This leads to sharp raise in CO levels followed by a drop in CO levels afternoon.
- The data set from location 2 shows a sudden drop in CO levels. This is due to the reason the sensor is placed near a open window. This allows more outdoor atmosphere to interact with the sensor. This concludes that CO levels in outdoor air is lower than indoor air. This research on comparison between indoor and Outdoor Air has some similar interesting observations.
- The temperature and humidity is inversely proportional to each other. Whenever there is a rise in temperature humidity drops and vice versa.
Algorithm:
- Take the required keys like API key, channel ID of Thing Speak, WiFi SSID and password as strings.
- Configure the analog and digital pins to take input.
- Wait for the device to connect to WiFi hotspot.
- Read and store the data from DHT11 (Temperature and Humidity) and MQ-07 then store it in a variable.
- Set the hardware to read sensor data per minute.
- Upload the data to cloud.
- Repeat the steps.
Code:
Download these libraries and move it in to your Arduino library folder. ESP8266, DHT-11 library and MQ-7. This project is built and tested by L Mrudula Qury. You can download all the files from GitHub repository here.
//Libraries #include <ESP8266WiFi.h> #include "dht.h" //for dht11 //Library for temperature and humidity sensor #include "MQ7.h" //for mq07 //Library for gas sensor //....Libraries end //DHT setup #define dht_apin D3 // Digital Pin sensor is connected to D3 of NodeMCU dht DHT; //...Setup end //Wifi setup and Thingspeak Credentials char* ssid="Your SSID"; //take wifi’s ssid char* pass="Your passwords"; //enter corresponding password char* host="api.thingspeak.com"; char* writeapikey="IIHQG7GURQAKBDDM"; // //...end //pin setup const int mq07=A0; //Mq07 connected to analog pin A0 of NodeMCU //...end //Mq7 setup MQ7 mq7(A0, 3.3); //As for nodeMCU vcc=3.3v //...end void setup() { Serial.begin(115200); WiFiClient client; //client variable //pinmodes pinMode(mq07,INPUT); //...end //WiFi connection WiFi.begin(ssid, pass); while(WiFi.status() != WL_CONNECTED) { delay(200); Serial.print(".."); } Serial.println(); Serial.println("NodeMCU is connected!"); Serial.println(WiFi.localIP()); //------------------------------------- } // varialbes required for project float Temperature,Humidity; float coquantity; const int httpport = 80; //--------------------------------------- void loop() { delay(2000); //temperature and humidity from DHT11 DHT.read11(dht_apin); Temperature=DHT.temperature; //loading temperature Humidity=DHT.humidity; //loading humidity Serial.print("Current humidity = "); Serial.print(Humidity); Serial.print("% "); Serial.print("temperature = "); Serial.print(Temperature); Serial.println("C "); //.....end //CO from mq07 coquantity = mq7.getPPM(); //reading COquantity in PPM Serial.print("COquantity : "); Serial.print(coquantity); Serial.println("ppm"); Serial.println("----------------------------------------------------"); //....end // client and pushing data to server (cloud) WiFiClient client; if(!client.connect(host,httpport)) { Serial.println("No Client"); return; } String url="/update?key="; url+=writeapikey; // uploding temperature data url+="&field1="; url+=String(Humidity); //--------------------------- // uploading humidity data url+="&field2="; url+=String(Temperature); //-------------------------- // uploading light intensity data url+="&field3="; url+=String(coquantity); //-------------------------- // uploading the url to cloud and disconnecting the client client.print(String("GET ") + url + "HTTP/1.1\r\n" + "Host : " + host + "\r\n" + "Connection: close\r\n\r\n"); client.stop(); //-------------------------- // Waiting for 60 seconds delay(60000); }
Share your feedback, queries and comments about this project in the comments section below. Check out other IOT projects in our website.